SpringMVC异常处理
系统中异常主要包括两部分,[编译时异常与运行时异常] Spring,SpringMVC,前者可以通过捕获异常从而获取异常信息,后者主要通过规范代码格式,测试等手段减少异常出现
在开发过程中,系统的DAO层,SERVICE层和CONTROLLER层都有可能出现异常情况,这种情况下我们应该尽量将异常向上层抛出,最后将所有异常交由SpringMVC的前端控制器处理,其会利用异常处理器来进行异常处理
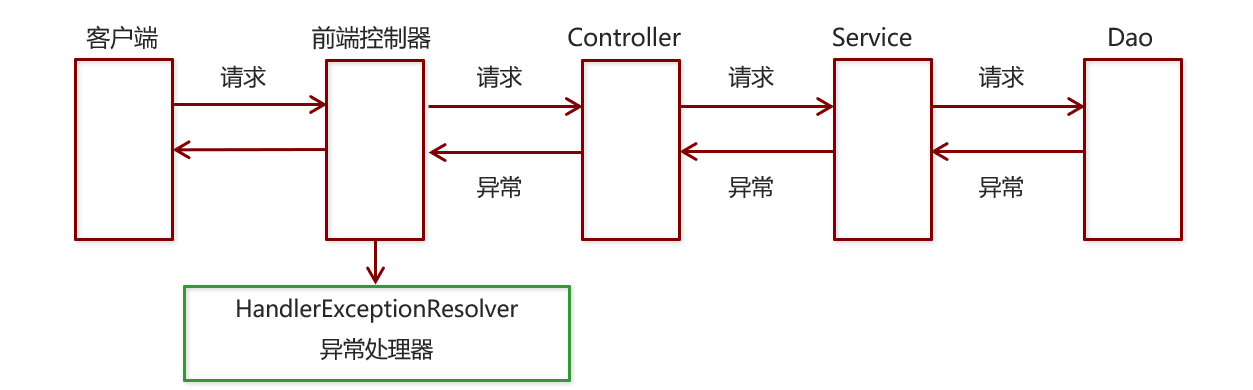
SpringMVC异常处理的两种方式
- 直接使用SpringMVC提供的简单异常处理器:SimpleMappingExceptionResolver
- 实现Spring的异常处理接口HandlerExceptionResolver自定义自己的异常处理器
1. 使用SimpleMappingExceptionResolver
配置简单映射异常处理器
1 2 3 4 5 6 7 8 9 10 11 12 13
| <bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver"> <property name="defaultErrorView" value="error"/> <property name="exceptionMappings"> <map> <entry key="cn.ywrby.exception.MyException" value="error"/> <entry key="java.lang.ClassCastException" value="error"/> </map> </property> </bean>
|
测试异常
产生异常的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| @Service("exceptionService") public class ExceptionServiceImpl implements ExceptionService { public void showError1(){ System.out.println("抛出类型转换异常"); Object str="Leslie"; Integer integer=(Integer)str; }
public void showError2(){ System.out.println("抛出除零异常"); int i=1/0; }
public void showError3() throws FileNotFoundException { System.out.println("抛出文件路径异常"); InputStream in=new FileInputStream("C:/xxx/xxx/xxx.txt"); }
public void showError4(){ System.out.println("抛出空指针异常"); String str=null; str.length(); }
public void showError5() throws MyException { System.out.println("抛出自定义异常"); throw new MyException(); } }
|
调用产生异常的方法
1 2 3 4 5 6 7 8 9 10 11
| @Controller("exceptionController") public class ExceptionController {
@Autowired private ExceptionService service;
@RequestMapping("/exception") public void testError(){ service.showError1(); } }
|
实例结果

2. 自定义异常处理器
创建异常处理器类实现HandlerExceptionResolver
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
public class MyExceptionResolver implements HandlerExceptionResolver { @Override public ModelAndView resolveException(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Object o, Exception e) { ModelAndView modelAndView=new ModelAndView(); if(e instanceof MyException){ modelAndView.addObject("info","自定义异常"); }else if(e instanceof ClassCastException){ modelAndView.addObject("info","类转换异常"); }else{ modelAndView.addObject("info","其他异常"); } modelAndView.setViewName("error"); return modelAndView; } }
|
配置异常处理器
1 2
| <bean class="cn.ywrby.resolver.MyExceptionResolver"/>
|
编写异常页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <%-- Created by IntelliJ IDEA. User: renboyu010214 Date: 2021/3/17 Time: 1:04 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Error1</title> </head> <body> <h1>Error:${info}</h1> </body> </html>
|
测试页面跳转
